Introduction
In this blog post, I will present the background processing framework (named bgPF in the rest of this post). Namely, I will talk about the basic idea behind it, list possible use-cases as well as the currently available features and show integration possibilities for monitoring purpose together with the available unit test environment. Of course, I will also demonstrate a simple coding example on how to use the bgPF.
The scope of this post is to present the main functionality of the bgPF without diving too deep into some more complex areas. Therefore, some of the more advanced features will only be named but not explained in detail.
Preconditions
- The bgPF is available available in the cloud (ABAP Environment for SAP BTP and SAP S/4HANA) and on premise starting with releases 2023
- To work with the bgPF, you need an inbound bgRFC destination. For cloud, no further action is needed, since this configuration has been performed by SAP for you.
For on-premise, you must create the default destination BGPF. Follow the steps below:
- Go to transaction SBGRFCCONF.
- Click on the tab Define Inbound Dest.
- Press the button with the tooltip Create.
On the Configure Inbound Destination dialog box, do the following.
- Enter the Inb. Dest. Name BGPF.
Do not enter information for Logon/Server Group or Add Queue Prefix.
- Choose Save.
Use case
The bgPF is a framework that offers functionality and convenience for applications, which need to run processing steps in the background, in a reliable way. It is a simple and easy-to-use feature to execute time-consuming ABAP methods asynchronously and reliably.
Consider, for example, a train booking application that has a screen for calculating the best and cheapest route to a destination. If you choose an offer, the application searches for activities you can do at the destination. Often, these applications redirect you to an activities screen where you still have to wait for the results. But what if you want to search for another destination instead of waiting?
That's exactly where the bgPF comes in place. You can realize a workflow, which searches for activities in the background while you're on to your next journey: The bgPF offers you the functionality to execute time-consuming methods asynchronously, if you do not need the result immediately. Instead, you can continue processing and come back to the result(s) later. Realization of such a scenario is massively eased by the bgPF.
Furthermore, the usage of the bgPF does also come in handy if you need to trigger functionality in a different session than your current one – e.g., because the functionality you want to call does a database commit while you are still in the interaction phase. This makes the bgPF a powerful tool in the ABAP RESTful Application Programming Model (RAP) and its transactional control.
Technical background (in a nutshell)
Just like you can use a car without having to know the laws of thermodynamics, you don’t need to know the technical background behind the bgPF to use it – promised!
So, if you are not interested and directly want to get your hands dirty, you can simply skip this part and go to the next one.
But let’s be honest: It’s much more fun if you know how things work
😊
The bgPF is built upon the background remote function call (bgRFC) but provides easy-to-use APIs that are completely abstracted from the bgRFC.
Furthermore, the bgPF offers RAP/ABAP cloud qualities like e.g., supportability, testability, typed APIs, ADT integration, debugging, extensibility, transactional consistency, … for usage in SAP BTP, ABAP Environment or SAP S/4HANA ABAP Environment.
Let’s look at below diagram for a general overview: The bgPF is used by an application to asynchronously call an application layer in a different ABAP application session via bgRFC:

In the synchronous session, the application creates an instance of the bgPF background process, which serializes the provided background operation. The serialized operation is used as input for the corresponding function method call, which in turn is written onto the bgRFC queue and saved for execution after a COMMIT WORK statement.
Therefore any referenced object must implement the IF_SERIALIZABLE_OBJECT interface, because otherwise it is not passed from the synchronous session to the asynchronous session when being serialized.
Once the asynchronous session is triggered via the bgRFC queue, the bgPF application process is deserialized and processed.
This process is also described in the following sequence diagram:

Available Features
Currently, you can use the following features:
- Transactional background processing
- Service Quality: Exactly once (i.e., the operations of a background process are executed exactly once without a specific order)
- A default inbound destination is part of the bgPF
- Support of Transactional Control in RAP
- Process for single Operation
- Exactly one operation can and must be added to this process
Examples
Using the bgPF with transactional control
If you are working with a RAP application and want to ensure transactional consistency by using the RAP transactional control (see
https://help.sap.com/docs/ABAP_PLATFORM_NEW/fc4c71aa50014fd1b43721701471913d/ccda1094b0f845e28b88f9f..., this scenario is the way to go. In the following, you will get to know the necessary steps.
As a reminder, in case of transactional control, the SAP LUW has two phases:
- Modify phase
- A consumer calls business object operations to change data and read instances with or without transactional changes. The business object runtime keeps the changes in its internal transactional buffer, which represents the state of the instance data. A transactional buffer is always required for a business object.
- Save phase
- After all changes were performed, data can be persisted.
Operation class
The operation class contains all the coding that shall be executed in the background session – i.e., on the asynchronous side. Let’s look at the coding for the transactional controlled operation:
CLASS cl_demo_bgpf_operation_contr DEFINITION
PUBLIC
FINAL
CREATE PUBLIC.
PUBLIC SECTION.
INTERFACES if_bgmc_op_single.
METHODS constructor
IMPORTING
iv_data TYPE string.
PRIVATE SECTION.
DATA mv_data TYPE string.
METHODS modify
RAISING
cx_bgmc_operation.
METHODS save.
ENDCLASS.
CLASS cl_demo_bgpf_operation_contr IMPLEMENTATION.
METHOD constructor.
mv_data = iv_data.
ENDMETHOD.
METHOD if_bgmc_op_single~execute.
modify( ).
cl_abap_tx=>save( ).
save( ).
ENDMETHOD.
METHOD modify.
IF mv_data IS INITIAL.
RAISE EXCEPTION NEW cx_demo_bgpf( textid = cx_demo_bgpf=>initial_input ).
ENDIF.
CONDENSE mv_data.
ENDMETHOD.
METHOD save.
MODIFY demo_bgpf FROM @( VALUE #( data = mv_data
changed_by = sy-uname ) ).
ENDMETHOD.
ENDCLASS.
In case of transactional control, your class must implement the interface IF_BGMC_OP_SINGLE. Our sample operation has input data, which is here handed over in the constructor, and shall be stored in the database table DEMO_BGPF with the following structure:
@EndUserText.label : 'Database table for bgPF'
@AbapCatalog.enhancement.category : #NOT_EXTENSIBLE
@AbapCatalog.tableCategory : #TRANSPARENT
@AbapCatalog.deliveryClass : #A
@AbapCatalog.dataMaintenance : #ALLOWED
define table demo_bgpf {
key client : abap.clnt not null;
data : abap.string(256);
@AbapCatalog.anonymizedWhenDelivered : false
changed_by : user;
}
Implementing IF_BGMC_OP_SINGLE~EXECUTE, the internal method MODIFY is called first, which checks if the input is initial. If it is, a background operation error is raised. The implementation also condenses the actual input. These actions are part of the transactional phase modify.
Please note:
Be careful when using member or global variable references in your operation. If a referenced object does not implement the IF_SERIALIZABLE_OBJECT interface, it is not passed from the synchronous session to the asynchronous session. Additionally, the constructor method of the operation is not called again in the asynchronous session.
Next, actions take place within the transactional phase save. First, we switch to save sequence by calling CL_ABAP_TX=>SAVE. Afterwards, the internal method SAVE is called and creates or modifies an entry in the database.
Starter class
The operation needs to be instantiated and handed over to the bgPF – this is the job of our starter class. This is the coding that is executed synchronously in the “original” ABAP application session and which triggers the background process. Let’s take a closer look at it:
CLASS cl_demo_bgpf_starter_contr DEFINITION
PUBLIC
INHERITING FROM cl_demo_classrun
CREATE PUBLIC.
PUBLIC SECTION.
METHODS main REDEFINITION.
PROTECTED SECTION.
PRIVATE SECTION.
ENDCLASS.
CLASS cl_demo_bgpf_starter_contr IMPLEMENTATION.
METHOD main.
DATA lo_operation TYPE REF TO if_bgmc_op_single.
DATA lo_process TYPE REF TO if_bgmc_process_single_op.
DATA lo_process_factory TYPE REF TO if_bgmc_process_factory.
DATA lo_process_monitor TYPE REF TO if_bgmc_process_monitor.
DATA lx_bgmc TYPE REF TO cx_bgmc.
lo_operation = NEW cl_demo_bgpf_operation_contr( 'Input data' ).
TRY.
lo_process_factory = cl_bgmc_process_factory=>get_default( ).
lo_process = lo_process_factory->create( ).
lo_process->set_name( 'Test process 1'
)->set_operation( lo_operation ).
lo_process_monitor = lo_process->save_for_execution( ).
COMMIT WORK.
CATCH cx_bgmc INTO lx_bgmc.
out->write( lx_bgmc->get_longtext( ) ).
ROLLBACK WORK.
ENDTRY.
ENDMETHOD.
ENDCLASS.
Please note:
If you are using the RAP runtime, COMMIT WORK or ROLLBACK WORK will be executed by the runtime. Do not initiate them with your own operation.
Starting off, an instance of the operation and an instance of the process factory for the default destination BGPF are created. The process factory is used to create an instance of a transactional background process for a single operation. After that the name of the process (here: Test process 1) is set and the operation is injected. The name of the process, for example, will be displayed in the ABAP Cross Trace – we will talk about this a little bit later. Finally, the process is saved for execution (returning an instance of the process monitor, which we will later have a closer look upon when talking about monitoring) and COMMIT WORK is executed. Without COMMIT WORK, the background process won't start. If an error occurs, an error text is displayed in the console and ROLLBACK WORK is executed to revert any changes done so far.
Tip
Inheriting from CL_DEMO_CLASSRUN you can simply execute your coding in the ABAP Development Tools via “Run As” > “ABAP Application (Console)” [Shortcut: F9].
Using the bgPF without transactional control
If you are not working with a RAP application (or do not need the strict transaction control), this scenario is the way to go. There are only some minor differences compared to our previous example:
- In contrast to the transactional controlled behavior, there is no need to differentiate between different transactional phases. All steps can be done at once.
- Instead of interface IF_BGMC_OP_SINGLE, your operation class must implement interface IF_BGMC_OP_SINGLE_TX_UNCONTR (and its EXECUTE method)
- In order to add an operation to the transactional background, you must use method SET_OPERATION_TX_UNCONTROLLED (instead of SET_OPERATION)
The rest of the coding is identical to the previous example.
Monitoring your background processes
It’s crucial to know what is going on - Knowledge is power!
But who you gonna call when you want to know the status of your background processes? Monitoring, of course! Which brings us directly to the topic:
There are two principal possibilities to monitor your background processes:
- Using the bgPF process monitor
- By integration of other SAP functionality
The bgPF process monitor
This is the approach for all those of you who want to do things by themselves!
The method IF_BGMC_PROCESS->SAVE_FOR_EXECUTION returns a monitor instance for the current background process, which can be used for your own accounting and monitoring.
The underlying interface - IF_BGMC_PROCESS_MONITOR – can be used to fetch the current state of the background process:
- If you are still in the same session that also saved the background process for execution, you can simply use method GET_STATE to fetch the current state of the background process – e.g., “New” or “Runnning” (compare to enum structure GCS_STATE in the interface)
- If you want to save the process monitor for a different session, use method TO_STRING and store the serialized string
- If you want to create an instance of the process monitor from a stored string, use method CL_BGMC_PROCESS_FACTORY=>CREATE_MONITOR_FROM_STRING together with the serialized string as input
Integration of other SAP functionality
Why invent the wheel again if there is already ready-to-use functionality?
It is possible to integrate the bgPF into other SAP functionality to monitor your background processes, namely:
- The bgRFC monitor (no further integration steps needed)
- The SAP Application Interface Framework (AIF) (only for OnPrem)
- The ABAP Cross-Tracer
- The ADT Performance Trace
Handling the bgRFC monitor and the integration into AIF is more complex and would go beyond the scope of this blog post, so we will focus on the ABAP Cross-Tracer and the ADT Performance Trace.
ABAP Cross-Tracer
To monitor your background projects via ABAP Cross-Tracer, we first need to create (via ADT view “ABAP Cross Trace” – section “Trace Configurations”) a new Trace Configuration and activate the Component
Background Processing (with ID
: SAP.BC.BGMC), as shown in the following figures:

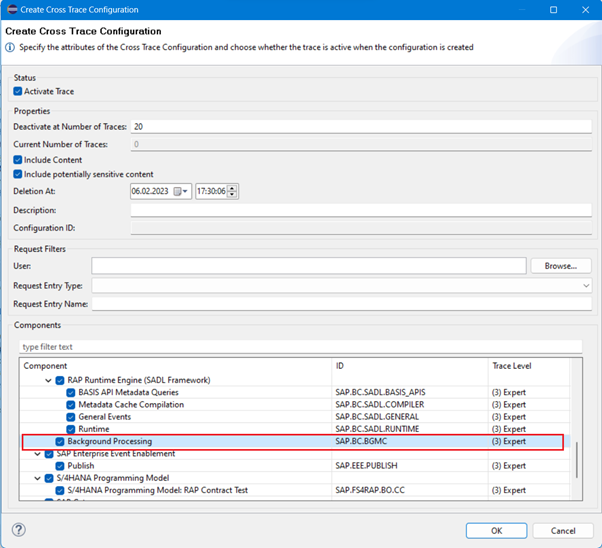
Example results (in ADT view “ABAP Cross Trace” – section “Trace Results”) can be seen below – the first entry is the saving of the background processing (method SAVE_FOR_EXECUTION) – i.e., the synchronous preparation of the background process -, the second entry is the asynchronous execution of the background process:
The first entry therefore only contains the information that the background process has been saved for execution:
The second entry contains the information that the execution of the background process has started and was completed successfully:
If an error during execution of the background process occurred, we would be able to see this in the corresponding entry (together with the underlying error message – “Operation failed” in this example):
In short, with the ABAP Cross-Trace, we can easily check the execution status of our background processes and see – if the execution failed – the corresponding exception text.
ADT Performance Trace
High performer or low performer - if you are interested in the performance of your background process, you can use the ADT Performance Trace to monitor this.
In order to create to create a corresponding trace configuration, switch to the ABAP Profiling view in your ADT and select the “ABAP Trace Requests” ADT View. Right click on your corresponding project and select “Create Trace Request”. In the editor, select
Remote Function Call (RFC) as type and filter for Function Module FM_BGMC_PROCESS:



Once your background process is finished, select ADT view “ABAP Traces” and check the second entry (the first call of the function module lies within method SAVE_FOR_EXECUTION of the background process instance – i.e., when the background process is prepared for execution - and is of no further interest here):
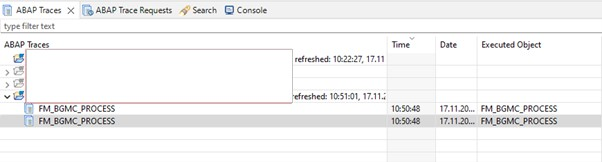
Within the resulting call sequence of the second entry, one can find the call of CL_BGMC_OPERATION_DECORATOR->IF_BGMC_OP_SINGLE~EXECUTE (or CL_BGMC_OPERATION_DECORATOR-> IF_BGMC_OP_SINGLE_TX_UNCONTR~EXECUTE for transactional uncontrolled operations) and the corresponding operations of the application, together with the corresponding performance data:

In short, the ADT Performance Trace can be utilized to examine the performance of your background process.
Writing Unit Tests for your bgPF implementation
It is always very neat to provide some unit tests for your coding – or even start with them when following the principles of test-driven development!
Therefore, the bgPF offers functionality which enables you to write unit tests for your synchronous bgPF coding: Using CL_BGMC_TEST_ENVIRONMENT=>CREATE_FOR_SPYING, you can create a test environment that offers functionality like asserting that the method SAVE_FOR_EXECUTION was called or that the number of created background processes is correct.
Let’s look at a simple example, where we will write a unit test for our bgPF-for-RAP example from the beginning:
CLASS test_demo_bgpf_starter_test DEFINITION FINAL FOR TESTING
DURATION SHORT
RISK LEVEL HARMLESS.
PRIVATE SECTION.
CLASS-DATA go_bgpf_test_env TYPE REF TO if_bgmc_test_envir_spy.
METHODS execute FOR TESTING RAISING cx_static_check.
METHODS teardown.
CLASS-METHODS class_setup.
CLASS-METHODS class_teardown.
ENDCLASS.
CLASS test_demo_bgpf_starter_test IMPLEMENTATION.
METHOD class_setup.
go_bgpf_test_env = cl_bgmc_test_environment=>create_for_spying( ).
ENDMETHOD.
METHOD class_teardown.
go_bgpf_test_env->destroy( ).
ENDMETHOD.
METHOD teardown.
go_bgpf_test_env->clear( ).
ENDMETHOD.
METHOD execute.
DATA lo_cut TYPE REF TO cl_demo_bgpf_starter_contr.
DATA lo_process_act TYPE REF TO if_bgmc_process_spy.
lo_cut = NEW cl_demo_bgpf_starter_contr ( ).
lo_cut->main( ).
lo_process_act = go_bgpf_test_env->assert_number_of_processes( 1
)->get_process( 1 ).
lo_process_act->assert_number_of_operations( 1
)->assert_is_saved_for_processing( ).
ENDMETHOD.
ENDCLASS.
The code does the following:
- CLASS_SETUP: An instance of the test environment for spying is created.
- CLASS_TEARDOWN: The test environment is destroyed.
- TEARDOWN: The test environment is cleared by removing all previously created processes from the test environment. Doing so, every unit test could be started with a new test environment.
Note:
Do not create several test environments at the same time. If you try to create a second test environment while there is another active environment an exception will be raised.
- EXECUTE: The actual unit test. An instance of the underlying bgPF starter (code under test) is created and executed.
- Assert that only one background process was created (IF_BGMC_PROCESS_FACTORY) and get an instance of the first (and only) process.
- Assert that the background process only contained one operation and that the method SAVE_FOR_EXECUTION of IF_BGMC_PROCESS_SINGLE_OP was called.
Note:
When using the test environment, the process method SAVE_FOR_EXECUTION does nothing, and the operations are not called. Instead, you can validate that the operations are instantiated and added to a process correctly. Operations need to be tested independently.
With this simple unit test, we have completely covered the synchronous side of our bgPF application and validated that our bgPF coding
- Created one background process
- Injected one background operation into our background process
- Successfully saved our background operation for execution
Summary
The background processing framework is a simple and easy-to-use feature to asynchronously and reliably execute ABAP methods. It is also a powerful tool in RAP (ABAP RESTful Application Programming Model) and its transactional control.
Monitoring possibilities using bgPF intrinsic as well as separate SAP solutions are available.
A unit test environment makes it simple to write unit tests for the (synchronous-side) bgPF coding.
Further reading
- For other options to asynchronously execute code using ABAP Cloud, see e.g. the following blog post
- The SAP Help Portal entry about the bgPF can be found here