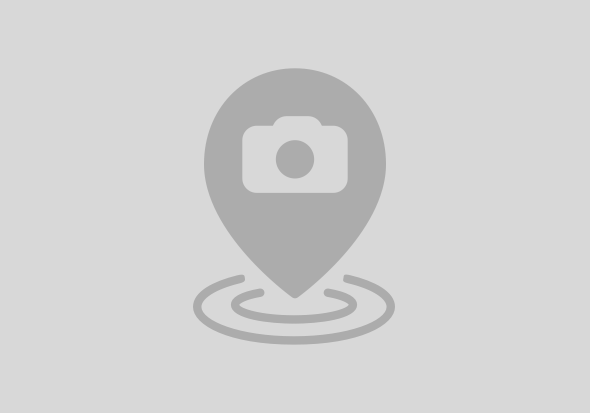
PART-1: UI5 APP
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.3.1</version>
</dependency>
@RestController
@RequestMapping("/v1/internal")
public class ImageMgmtService {
final static Logger LOGGER = LoggerFactory.getLogger(ImageMgmtServiceImpl.class);
private ImageMgmtServiceImpl imageServiceImpl = new ImageMgmtServiceImpl();
EntityManagerFactory emf ;
@InitBinder
public void initBinder(WebDataBinder dataBinder){
dataBinder.setDisallowedFields();
}
@PersistenceUnit
public void setEntityManagerFactory(EntityManagerFactory emf) {
this.emf = emf; }
@RequestMapping(value = "/uploadImage", method = RequestMethod.POST, produces = MediaType.APPLICATION_JSON_VALUE)
public ResponseEntity<ImageManagementServiceResponse> getuploadImage(@RequestParam("file") MultipartFile file,
@RequestParam("file-data") String data) throws JSONException {
JSONObject obj = new JSONObject(data);
Integer mappingGuid = (Integer) obj.get("mapping_Guid");
return imageServiceImpl.uploadImage(file, mappingGuid , emf);
}
@RequestMapping(value = "/fetchImage/{guid}", method = RequestMethod.GET)
public ResponseEntity fetchImage(@PathVariable("guid") String mappingGuid )
{
return imageServiceImpl.fetchImage(mappingGuid, emf);}
}
public class ImageMgmtServiceImpl {
final static Logger LOGGER = LoggerFactory.getLogger(ImageMgmtServiceImpl.class);
HttpStatus statusCode = null;
EntityManagerFactory emf;
public ResponseEntity<ImageManagementServiceResponse> uploadImage(MultipartFile file, Integer mappingGUID,
EntityManagerFactory emf) {
this.emf = emf;
return doUploadImage(file, mappingGUID);
}
private ResponseEntity<ImageManagementServiceResponse> doUploadImage(MultipartFile file, Integer mappingGuid) {
EntityManager em = this.emf.createEntityManager();
HttpHeaders httpHeaders = new HttpHeaders();
ImageManagementServiceResponse response = null;
try {
String EVENT_IDENTFIER = "com.sap.sample::IMAGE_MANAGEMENT.IMAGE_STORE";
String eventIdentificationCall = "INSERT INTO \"" + EVENT_IDENTFIER + "\" VALUES (?,?,?)";
em.getTransaction().begin();
Query query = em.createNativeQuery(eventIdentificationCall);
query.setParameter(1, mappingGuid);
query.setParameter(2, file.getContentType());
query.setParameter(3, file.getBytes());
int records = query.executeUpdate();
LOGGER.info("The image has been uploaded" + records);
em.getTransaction().commit();
response = new ImageManagementServiceResponse();
response.setGuid(mappingGuid + "");
response.setLevel("1");
response.setSuccess(true);
return new ResponseEntity<ImageManagementServiceResponse>(response, httpHeaders, HttpStatus.OK);
} catch (Exception ex) {
if ((em != null) && (em.isOpen() == true)) {
em.close();
}
response = new ImageManagementServiceResponse();
response.setGuid(mappingGuid + "");
response.setLevel("0");
response.setSuccess(false);
response.setReasonType(0);
response.setReasonDescription("Image cannot be uploaded");
LOGGER.error("tenantName: " + ",Upload image failed : " + ex.getMessage());
return new ResponseEntity<ImageManagementServiceResponse>(response, httpHeaders, HttpStatus.OK);
} finally {
if (em != null) {
em.close();
}
}
}
public ResponseEntity fetchImage(String guid, EntityManagerFactory emf) {
return doFetchImage(guid, emf);
}
private ResponseEntity doFetchImage(String guid, EntityManagerFactory emf) {
EntityManager em = emf.createEntityManager();
em.getTransaction().begin();
try {
String fetchCall = "com.sap.sample::IMAGE_MANAGEMENT.IMAGE_STORE";
String eventIdentificationCall = "SELECT * FROM \"" + fetchCall + "\" WHERE IMAGE_ID = ?";
Query query = em.createNativeQuery(eventIdentificationCall, ImageOutput.class);
query.setParameter(1, guid);
ImageOutput imo = (ImageOutput) query.getSingleResult();
byte[] image = imo.getImageBinary();
HttpHeaders httpHeaders = new HttpHeaders();
httpHeaders.add("Content-Type", imo.getImageMime());
httpHeaders.add("Cache-Control", "max-age=259200");
em.getTransaction().commit();
return new ResponseEntity(image, httpHeaders, HttpStatus.OK);
} catch (Exception ex) {
try {
File file = new File(getClass().getClassLoader().getResource("default-image.png").getFile());
HttpHeaders httpHeaders = new HttpHeaders();
httpHeaders.add("Content-Type", "image/png");
byte[] image = IOUtils.toByteArray(new FileInputStream(file));
LOGGER.error("tenantName: " + ",Upload fetch failed : " + ex.getMessage());
return new ResponseEntity(image, httpHeaders, HttpStatus.OK);
} catch (Exception e) {
HttpHeaders httpHeaders = new HttpHeaders();
LOGGER.error("tenantName: " + ",Upload fetch failed : " + ex.getMessage());
return new ResponseEntity(httpHeaders, HttpStatus.BAD_REQUEST);
}
}
}
}
public class ImageManagementServiceResponse {
private byte[] image;
private String level;
private String guid;
private Boolean success;
private Integer reasonType;
private String reasonDescription;
private Object data;
// setter and getter method needs to be generated here
}
@Entity
@Table(name="\"com.sap.sample::IMAGE_MANAGEMENT.IMAGE_STORE\"")
public class ImageStorage implements Serializable{
private static final long serialVersionUID = 4829386985899147977L;
@Id
@Column(name="IMAGE_ID")
private String imageId = null;
@Lob
@Column(name = "IMAGE_BINARY")
private byte[] imageBinary = null;
@Column(name = "IMAGE_MIME_TYPE")
private String imageMimeType = null;
public String getImageId() {
return imageId;
}
public void setImageId(String imageId) {
this.imageId = imageId;
}
public String getImageMimeType() {
return imageMimeType;
}
public void setImageMimeType(String imageMimeType) {
this.imageMimeType = imageMimeType;
}
public static long getSerialversionuid() {
return serialVersionUID;
}
public byte[] getImageBinary() {
return imageBinary;
}
public void setImageBinary(byte[] imageBinary) {
this.imageBinary = imageBinary;
}
Few of my other blogs:-
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
10 | |
9 | |
8 | |
7 | |
7 | |
6 | |
6 | |
5 | |
5 | |
5 |