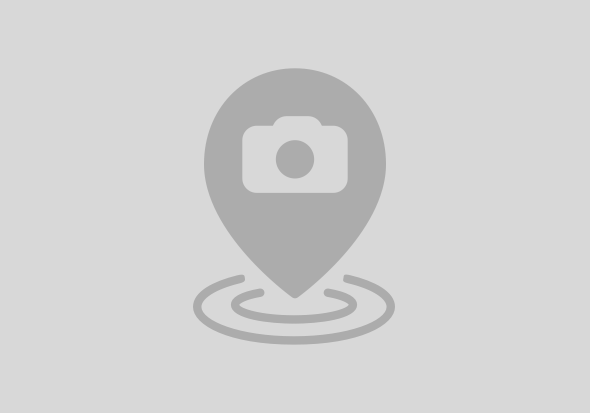
FUNCTION STFC_STRUCTURE.
*"----------------------------------------------------------------------
*"*"Lokale Schnittstelle:
*" IMPORTING
*" VALUE(IMPORTSTRUCT) LIKE RFCTEST STRUCTURE RFCTEST
*" EXPORTING
*" VALUE(ECHOSTRUCT) LIKE RFCTEST STRUCTURE RFCTEST
*" VALUE(RESPTEXT) LIKE SY-LISEL
*" TABLES
*" RFCTABLE STRUCTURE RFCTEST
*"----------------------------------------------------------------------
function my_stfc_structure(request_context, abap_input) {
// inspect request context
const attributes = request_context["connection_attributes"];
console.log(
"[js] my_stfc_structure context:",
attributes["sysId"], attributes["client"], attributes["user"], attributes["progName"]);
console.log("[js] my_stfc_structure input:", abap_input.IMPORTSTRUCT);
// prepare response for ABAP client
const echostruct = abap_input.IMPORTSTRUCT;
echostruct.RFCINT1 = 2 * echostruct.RFCINT1;
echostruct.RFCINT2 = 3 * echostruct.RFCINT2;
echostruct.RFCINT4 = 4 * echostruct.RFCINT4;
const abap_output = {
ECHOSTRUCT: echostruct,
RESPTEXT: `~~~ Node server here ~~~`,
};
console.log("[js] my_stfc_structure response:", abap_output);
// return response data
return abap_output;
}
call function 'STFC_STRUCTURE' destination 'NWRFC_SERVER_OS'
exporting
importstruct = ls_struct
importing
echostruct = ls_struct
resptext = lv_resp
tables
rfctable = lt_table
exceptions
communication_failure = 1 message lv_error_message
system_failure = 2 message lv_error_message.
RFC destination configuration - technical settings
RFC destinatin configuration - bgRFC support
DEST=MME
USER=demo
PASSWD=welcome
ASHOST=system51
SYSNR=00
CLIENT=620
LANG=EN
TRACE=0
DEST=MME_GATEWAY
GWSERV=sapgw00
GWHOST=coevi51
PROGRAM_ID=RFCSERVER
REG_COUNT=1
mkdir server
cd server
npm init -y
npm install node-rfc
import {RfcLoggingLevel, Server} from "node-rfc";
// Create server instance, initially inactive
const server = new Server({
serverConnection: { dest: "MME_GATEWAY" },
clientConnection: { dest: "MME" },
// Server options are optional
serverOptions: {
logLevel: RfcLoggingLevel.error,
// authHandler: authHandler,
},
});
(async () => {
try {
// Start the server
await server.start();
console.log(
`[js] Server alive: ${server.alive} client handle: ${server.client_connection}`,
`server handle: ${server.server_connection}`
);
} catch (ex) {
// Catch errors, if any
console.error(ex);
}
})();
// Close the server after 10 seconds
let seconds = 10;
const tick = setInterval(() => {
console.log("tick", --seconds);
if (seconds <= 0) {
server.stop(() => {
clearInterval(tick);
console.log("bye!");
});
}
}, 1000);
RFC destination - connection test
RFC destination connection test output
// Server function
function my_stfc_structure(request_context, abap_input) {
const connection_attributes = request_context["connection_attributes"];
console.log(
"[js] my_stfc_structure context:",
connection_attributes["sysId"],
connection_attributes["client"],
connection_attributes["user"],
connection_attributes["progName"]
);
console.log("[js] my_stfc_structure input:", abap_input.IMPORTSTRUCT);
const echostruct = abap_input.IMPORTSTRUCT;
echostruct.RFCINT1 = 2 * echostruct.RFCINT1;
echostruct.RFCINT2 = 3 * echostruct.RFCINT2;
echostruct.RFCINT4 = 4 * echostruct.RFCINT4;
const abap_output = {
ECHOSTRUCT: echostruct,
RESPTEXT: `~~~ Node server here ~~~`,
};
console.log("[js] my_stfc_structure response:", abap_output);
return abap_output;
}
server.addFunction("STFC_STRUCTURE", my_stfc_structure);
import { RfcLoggingLevel, Server } from "node-rfc";
// Create server instance, initially inactive
const server = new Server({
serverConnection: { dest: "MME_GATEWAY" },
clientConnection: { dest: "MME" },
// Server options are optional
serverOptions: {
logLevel: RfcLoggingLevel.error,
// authHandler: authHandler,
},
});
// Server function
function my_stfc_structure(request_context, abap_input) {
const connection_attributes = request_context["connection_attributes"];
console.log(
"[js] my_stfc_structure context:",
connection_attributes["sysId"],
connection_attributes["client"],
connection_attributes["user"],
connection_attributes["progName"]
);
console.log("[js] my_stfc_structure input:", abap_input.IMPORTSTRUCT);
const echostruct = abap_input.IMPORTSTRUCT;
echostruct.RFCINT1 = 2 * echostruct.RFCINT1;
echostruct.RFCINT2 = 3 * echostruct.RFCINT2;
echostruct.RFCINT4 = 4 * echostruct.RFCINT4;
const abap_output = {
ECHOSTRUCT: echostruct,
RESPTEXT: `~~~ Node server here ~~~`,
};
console.log("[js] my_stfc_structure response:", abap_output);
return abap_output;
}
(async () => {
try {
// Register server function
server.addFunction("STFC_STRUCTURE", my_stfc_structure);
console.log(
`[js] Node.js function '${my_stfc_structure.name}'`,
"registered as ABAP 'STFC_STRUCTURE' function"
);
// Start the server
await server.start();
console.log(
`[js] Server alive: ${server.alive} client handle: ${server.client_connection}`,
`server handle: ${server.server_connection}`
);
} catch (ex) {
// Catch errors, if any
console.error(ex);
}
})();
// Close the server after 10 seconds
let seconds = 10;
const tick = setInterval(() => {
console.log("tick", --seconds);
if (seconds <= 0) {
server.stop(() => {
clearInterval(tick);
console.log("bye!");
});
}
}, 1000);
ts-node ci/test/server-test.ts (py3.11.4) ✘ 1 main ◼
[js] Node.js function 'my_stfc_structure' registered as ABAP 'STFC_STRUCTURE' function
[js] Server alive: false client handle: 5554800128 server handle: 0
tick 9
tick 8
[js] my_stfc_structure context: MME 620 D037732 ZSERVER_STFC_STRUCT
[js] my_stfc_structure input: {
RFCFLOAT: 0,
RFCCHAR1: '',
RFCINT2: 2,
RFCINT1: 1,
RFCCHAR4: '',
RFCINT4: 4,
RFCHEX3: <Buffer 00 00 00>,
RFCCHAR2: '',
RFCTIME: '000000',
RFCDATE: '00000000',
RFCDATA1: '',
RFCDATA2: ''
}
[js] my_stfc_structure response: {
ECHOSTRUCT: {
RFCFLOAT: 0,
RFCCHAR1: '',
RFCINT2: 6,
RFCINT1: 2,
RFCCHAR4: '',
RFCINT4: 16,
RFCHEX3: <Buffer 00 00 00>,
RFCCHAR2: '',
RFCTIME: '000000',
RFCDATE: '00000000',
RFCDATA1: '',
RFCDATA2: ''
},
RESPTEXT: '~~~ Node server here ~~~'
}
tick 7
tick 6
tick 5
^C
*&---------------------------------------------------------------------*
*& Report ZSERVER_STFC_STRUCT
*&---------------------------------------------------------------------*
*&
*&---------------------------------------------------------------------*
report zserver_stfc_struct.
data lv_echo like sy-lisel.
data lv_resp like sy-lisel.
data ls_struct like rfctest.
data lt_table like table of rfctest.
data lv_error_message type char512.
ls_struct-rfcint1 = 1.
ls_struct-rfcint2 = 2.
ls_struct-rfcint4 = 4.
insert ls_struct into table lt_table.
call function 'STFC_STRUCTURE' destination 'NWRFC_SERVER_OS'
exporting
importstruct = ls_struct
importing
echostruct = ls_struct
resptext = lv_resp
tables
rfctable = lt_table
exceptions
communication_failure = 1 message lv_error_message
system_failure = 2 message lv_error_message.
if sy-subrc eq 0.
write: / 'rfcint1:', ls_struct-rfcint1.
write: / 'rfcint2:', ls_struct-rfcint2.
write: / 'rfcint4:', ls_struct-rfcint4.
write: / 'resptext:', lv_resp.
else.
write: 'subrc :', sy-subrc.
write: / 'msgid :', sy-msgid, sy-msgty, sy-msgno.
write: / 'msgv1-4:', sy-msgv1, sy-msgv2, sy-msgv3, sy-msgv4.
write: / 'message:', lv_error_message.
exit.
endif.
Node.js server call output
throw new Error("my_stfc_function error");
console.log("[js] my_stfc_structure response:", abap_output);
return abap_output;
}
ABAP test report - error
Error log
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
21 | |
14 | |
13 | |
11 | |
10 | |
10 | |
9 | |
9 | |
8 | |
7 |