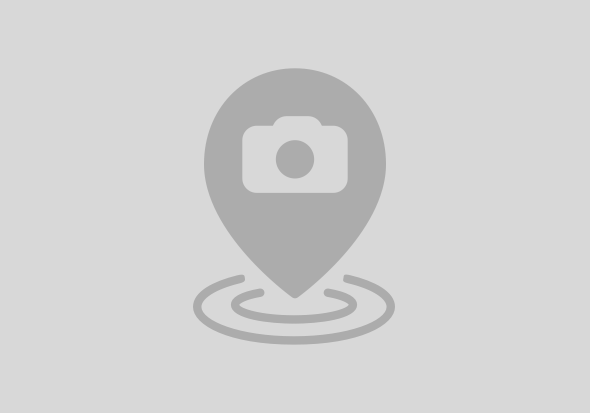
npm init @ui5/webcomponents-package@latest
<div class="star-wars-intro">
<p class="intro-text">{{intro}}</p>
<h2 class="main-logo">
<img src="{{logo}}"/>
</h2>
<div class="main-content">
<div class="title-content">
<slot></slot>
</div>
</div>
</div>
import slot from "@ui5/webcomponents-base/dist/decorators/slot.js";
/**
* Defines the intro of the space component.
*
* @type {string}
* @name demo.components.SpaceComponent.prototype.intro
* @defaultvalue ""
* @public
*/
@property()
intro!: string;
/**
* Defines the logo of the space component.
*
* @type {string}
* @name demo.components.SpaceComponent.prototype.logo
* @defaultvalue ""
* @public
*/
@property()
logo!: string;
/**
* Defines the articles of the component.
*
* @type {demo.components.SpaceItemComponent[]}
* @name demo.components.SpaceComponent.prototype.default
* @slot items
* @public
*/
@slot({ type: HTMLElement, "default": true })
items!: Array<SpaceItemComponent>;
onAfterRendering() {
const numStars = 100;
const mainDiv = this.shadowRoot!.querySelector(".star-wars-intro") as HTMLElement;
// For every star we want to display
for (let i = 0; i < numStars; i++) {
const { top, left } = this.getRandomPosition(mainDiv);
mainDiv.append(this.getRandomStar(top, left));
}
}
getRandomStar(top: string, left: string) {
const star = document.createElement("div");
star.className = "star";
star.style.top = top;
star.style.left = left;
return star;
}
getRandomPosition(element: HTMLElement) {
return {
top: `${this.getRandomNumber(element.offsetHeight)}px`,
left: `${this.getRandomNumber(element.offsetWidth)}px`,
};
}
getRandomNumber(value: number) {
return Math.floor(Math.random() * value);
}
npm run create-ui5-element
import "./dist/SpaceItemComponent.js";
<article class="space-article">
<h2>{{title}}</h2>
<p>{{description}}</p>
</article>
import UI5Element from "@ui5/webcomponents-base/dist/UI5Element.js";
import customElement from "@ui5/webcomponents-base/dist/decorators/customElement.js";
import litRender from "@ui5/webcomponents-base/dist/renderer/LitRenderer.js";
import { getI18nBundle } from "@ui5/webcomponents-base/dist/i18nBundle.js";
import type I18nBundle from "@ui5/webcomponents-base/dist/i18nBundle.js";
import property from "@ui5/webcomponents-base/dist/decorators/property.js";
// Template
import SpaceItemComponentTemplate from "./generated/templates/SpaceItemComponentTemplate.lit.js";
// Styles
import SpaceItemComponentCss from "./generated/themes/SpaceItemComponent.css.js";
/**
* @class
*
* <h3 class="comment-api-title">Overview</h3>
*
*
* <h3>Usage</h3>
*
* For the <code>ui5-space-item-component</code>
* <h3>ES6 Module Import</h3>
*
* <code>import SpacePackage/dist/SpaceItemComponent.js";</code>
*
* @constructor
* @alias demo.components.SpaceItemComponent
* @extends sap.ui.webc.base.UI5Element
* @tagname space-item-component
* @public
*/
@customElement({
tag: "space-item-component",
renderer: litRender,
styles: SpaceItemComponentCss,
template: SpaceItemComponentTemplate,
})
class SpaceItemComponent extends UI5Element {
/**
* Defines the title of the space item component.
*
* @type {string}
* @name demo.components.SpaceItemComponent.prototype.title
* @defaultvalue ""
* @public
*/
@property()
title!: string;
/**
* Defines the description of the space item component.
*
* @type {string}
* @name demo.components.SpaceItemComponent.prototype.description
* @defaultvalue ""
* @public
*/
@property()
description!: string;
}
SpaceItemComponent.define();
export default SpaceItemComponent;
.space-article>h2 {
text-align: center;
}
import SpaceItemComponent from "./SpaceItemComponent.js";
<space-component id="myFirstComponent" intro="Hello UI5con!" logo="./img/star-wars-intro.png">
<space-item-component title="EPISODES IV-VI" description="After years of galactic silence, civilization is on the brink of a new Star Wars release. Now, with the Force preparing to awaken, the people of Earth seek solace in films of old. With nowhere to turn, they gather in great numbers and watch the original trilogy without rest. Three films. 6 hours. 24 minutes. Popcorn. Slushies. Total elation."></space-item-component>
<space-item-component title="Episode V: The Empire Strikes Back Opener" description="It is a dark time for the Rebellion. Although the Death Star has been destroyed, Imperial troops have driven the Rebel forces from their hidden base and pursued them across the galaxy.Evading the dreaded Imperial Starfleet, a group of freedom fighters led by Luke Skywalker has established a new secret base on the remote ice world of Hoth.The evil lord Darth Vader, obsessed with finding young Skywalker, has dispatched thousands of remote probes into the far reaches of space"></space-item-component>
</space-component>
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
8 | |
7 | |
5 | |
4 | |
4 | |
4 | |
4 | |
4 | |
3 | |
3 |