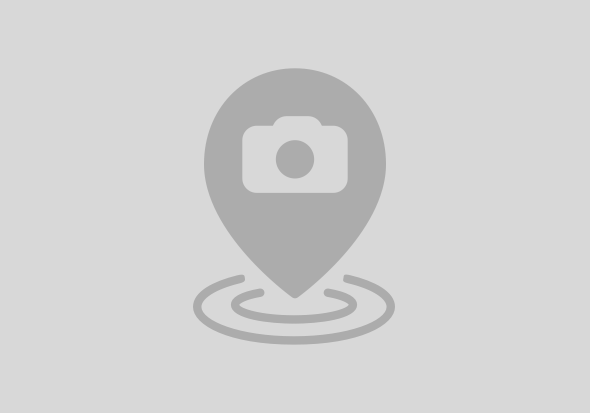
Hello, this is my first ABAP article. In this article, I will discuss String Template, a feature in ABAP code that simplifies string representation.
It all began with a request to change the output of the BAPI result accessed from non-SAP systems. The amount value in the BAPI result had already been converted to the char data type. The output amount at that time followed the SAP number format. For negative numbers, the minus sign was placed behind the number. Thus, Functionals requested a change for it.
TYPES: BEGIN OF ty_dec,
name TYPE sgtxt,
price TYPE wrbtr,
END OF ty_dec.
DATA: li_dec TYPE TABLE OF ty_dec.
li_dec = VALUE #(
( name = 'Item 01' price = '112.00' )
( name = 'Item 02' price = '89.09' )
( name = 'Item 03' price = '31.53-' )
( name = 'Item 04' price = '102.23' )
).
"-- show initial data
WRITE: / 'Initial Result:'.
LOOP AT li_dec INTO DATA(wa_dec).
DATA(lv_price) = CONV char30( wa_dec-price ).
CONDENSE lv_price.
WRITE: / wa_dec-name, lv_price.
ENDLOOP.
Initial result from the original request
Armed with superficial knowledge, I attempted to modify the output value of the char data type using ABAP substring. It turned out to work well, but it required a “few” lines of code and a temporary variable to accomplish. However, for more experienced and clever programmers, it probably could be done in fewer lines and without any temporary variables, my bad.
"-- result of conversion #1
WRITE: / 'Conversion #1:'.
LOOP AT li_dec INTO wa_dec.
lv_price = CONV char30( wa_dec-price ).
CONDENSE lv_price.
IF wa_dec-price < 0.
DATA(lv_len) = strlen( lv_price ) - 1.
lv_price = |{ lv_price+lv_len(1) }{ lv_price(lv_len) }|.
WRITE: / wa_dec-name, lv_price.
ELSE.
WRITE: / wa_dec-name, lv_price.
ENDIF.
ENDLOOP.
The minus sign was moved to the front of the number
Later, while coincidentally browsing the internet, I discovered the string template feature with embedded expressions in ABAP that is compatible with my current system, version 7.56. It only required 1 line of code to move the minus sign from behind to the front of the number. No substring or Function Module was needed.
"-- result of conversion #2
WRITE: / 'Conversion #2:'.
LOOP AT li_dec INTO wa_dec.
lv_price = |{ wa_dec-price SIGN = LEFT }|.
WRITE: / wa_dec-name, lv_price.
ENDLOOP.
The minus sign was moved to the front of the number, but more efficient
This article serves as a reminder to myself that many simple features in ABAP codes create efficient code, which I hadn’t been aware of before. And also, I hope it will help fellow ABAP programmers.
For more about the String template, follow the link for complete documentation.
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
9 | |
8 | |
5 | |
5 | |
4 | |
4 | |
4 | |
3 | |
3 | |
3 |