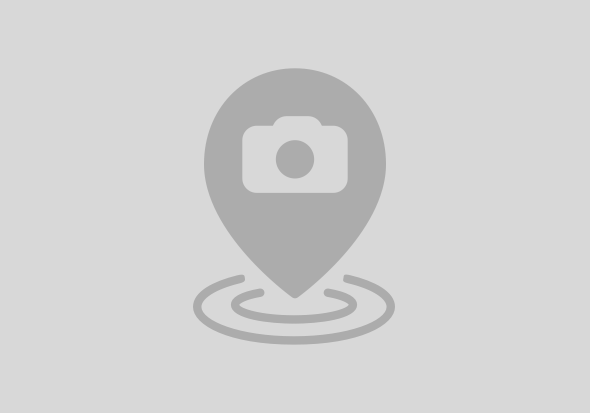
package com.sap.cookie.validation;
public class ResponseModel {
private String User;
private String Sysid;
private String Client;
private String PrtUsr;
private String SubjectDN;
private String IssuerDN;
private int errorCode;
private String errorMessage;
public ResponseModel(String User, String Sysid, String Client, String PrtUsr) {
this.setUser(User);
this.setSysid(Sysid);
this.setClient(Client);
this.setPrtUsr(PrtUsr);
}
public ResponseModel(int errorCode, String errorMessage) {
this.setErrorCode(errorCode);
this.setErrorMessage(errorMessage);
}
public String getUser() {
return User;
}
public void setUser(String user) {
User = user;
}
public String getSysid() {
return Sysid;
}
public void setSysid(String sysid) {
Sysid = sysid;
}
public String getClient() {
return Client;
}
public void setClient(String client) {
Client = client;
}
public String getPrtUsr() {
return PrtUsr;
}
public void setPrtUsr(String prtUsr) {
PrtUsr = prtUsr;
}
public int getErrorCode() {
return errorCode;
}
public void setErrorCode(int errorCode) {
this.errorCode = errorCode;
}
public String getErrorMessage() {
return errorMessage;
}
public void setErrorMessage(String errorMessage) {
this.errorMessage = errorMessage;
}
public String getSubjectDN() {
return SubjectDN;
}
public void setSubjectDN(String subjectDN) {
SubjectDN = subjectDN;
}
public String getIssuerDN() {
return IssuerDN;
}
public void setIssuerDN(String issuerDN) {
IssuerDN = issuerDN;
}
}
package com.sap.cookie.validation;
import java.io.ByteArrayInputStream;
import java.security.cert.CertificateFactory;
import java.security.cert.X509Certificate;
import java.util.Base64;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.mysap.sso.SSO2Ticket;
public class Ticket {
public String decode(String sapCookie, String certificate) {
Gson gson = new GsonBuilder().serializeNulls().create();
ResponseModel response = null;
Object[] o = null;
String code = "";
try {
// Decode Base64 Certificate into Array of bytes
byte[] decodedBytes = Base64.getDecoder().decode(certificate);
// Load Certificate into memory
boolean result = SSO2Ticket.loadKey(decodedBytes, null, 0, 1);
if (result == false) {
// Add error code and error message to response
response = new ResponseModel(500, "Certificate failed to load into memory!");
// Return error response in JSON
return gson.toJson(response);
}
// Call function to decrypt a given MYSAPSSO2 Cookie. No need to pass the
// certificate here as it was loaded into memory on the step before
o = SSO2Ticket.evalLogonTicket(sapCookie, null, null);
if (o != null) {
// Decryption was successful, create response with user details retrieved from
// cookie object
response = new ResponseModel(o[0].toString(), o[1].toString(), o[2].toString(), o[4].toString());
// The third element is the certificate in byte
// representation, to get its contents we need
// to first convert it into a cert structure
byte[] cert_;
X509Certificate cert = null;
if (o.length > 3) {
cert_ = (byte[]) o[3];
CertificateFactory cf = CertificateFactory.getInstance("X.509");
cert = (X509Certificate) cf.generateCertificate(new ByteArrayInputStream(cert_));
// Add certificate details to response
response.setSubjectDN(cert.getSubjectDN().toString());
response.setIssuerDN(cert.getIssuerDN().toString());
} else {
cert = null;
}
}
} catch (Exception e) {
String error = e.getMessage();
// Get error code from exception message
code = error.substring(47, error.lastIndexOf(','));
String errorMessage = "";
// Map error code to relevant error message
switch (code) {
case "1":
errorMessage = "No user provided to function";
break;
case "3":
errorMessage = "Provided buffer too small";
break;
case "4":
errorMessage = "Ticket expired";
break;
case "5":
errorMessage = "Ticket syntactically invalid";
break;
case "6":
errorMessage = "provided buffer too small";
break;
case "8":
errorMessage = "no ticket provided to func";
break;
case "9":
errorMessage = "Internal error";
break;
case "11":
errorMessage = "malloc faile";
break;
case "12":
errorMessage = "wrong action";
break;
case "13":
errorMessage = "tried to call NULL p. function";
break;
case "14":
errorMessage = "Error occured in security tk";
break;
case "15":
errorMessage = "pointer was NULL";
break;
case "16":
errorMessage = "incomplete information in t.";
break;
case "17":
errorMessage = "couldn’t get certificate";
break;
case "20":
errorMessage = "Signature couldn’t be verified";
break;
case "21":
errorMessage = "Ticket too new for lib";
break;
case "22":
errorMessage = "Conversion error";
break;
case "23":
errorMessage = "Recipient missing";
break;
case "24":
errorMessage = "Recipient different";
break;
default:
errorMessage = "Decryption default error";
}
// Add error code and error message to response
response = new ResponseModel(Integer.parseInt(code), errorMessage);
}
// Return response in JSON
return gson.toJson(response);
}
}
/* Refer the link below to learn more about the use cases of script.
https://help.sap.com/viewer/368c481cd6954bdfa5d0435479fd4eaf/Cloud/en-US/148851bf8192412cba1f9d2c17f...
If you want to know more about the SCRIPT APIs, refer the link below
https://help.sap.com/doc/a56f52e1a58e4e2bac7f7adbf45b2e26/Cloud/en-US/index.html */
import com.sap.gateway.ip.core.customdev.util.Message;
import java.nio.charset.StandardCharsets;
def Message parse(Message message) {
String query = message.getHeader('CamelHttpQuery', String)
query = (query) ? URLDecoder.decode(query, StandardCharsets.UTF_8.name()) : ''
Map<String, String> queryParameters = query.tokenize('&').collectEntries { entry ->
entry.tokenize('=').toSpreadMap().collectEntries { parameter ->
['QueryParameter_' + parameter.key, parameter.value]
}
}
message.setProperties(queryParameters)
return message;
}
/* Refer the link below to learn more about the use cases of script.
https://help.sap.com/viewer/368c481cd6954bdfa5d0435479fd4eaf/Cloud/en-US/148851bf8192412cba1f9d2c17f...
If you want to know more about the SCRIPT APIs, refer the link below
https://help.sap.com/doc/a56f52e1a58e4e2bac7f7adbf45b2e26/Cloud/en-US/index.html */
import com.sap.gateway.ip.core.customdev.util.Message;
import java.util.HashMap;
import com.mysap.sso.SSO2Ticket;
import com.sap.cookie.decryption.Ticket;
def Message processData(Message message) {
//Properties
def properties = message.getProperties();
def sapCookie = properties.get("QueryParameter_SapCookie");
def certificate = properties.get("QueryParameter_Certificate");
Ticket ticket = new Ticket();
def result = ticket.decode(sapCookie,certificate);
message.setBody(result);
message.setHeader("Content-Type", "application/json" + "; charset=utf-8" );
return message;
}
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
11 | |
6 | |
5 | |
5 | |
4 | |
4 | |
4 | |
3 | |
3 | |
3 |