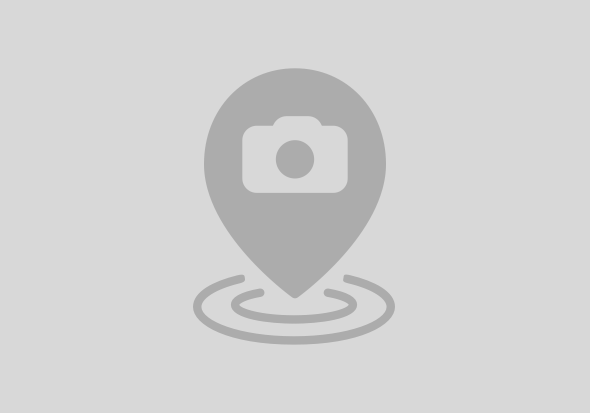
Bring up the UI with ctrl+alt+shift+T
ResourceModel
can be used to alter the way i18n translations worked.ctrl+alt+shift+T
the menu appears. On the menu simple settings are made to change the output the resource model provides. What the resource and the user interface does is completely transparent from the point of view of the host application.ctrl+alt+shift+T
sap/ui/core/Popup
which allows the creation of popup elements independently of other controls. I created a popup then assigned a Fragment to it. To avoid external file operations the fragment was defined inside the JS code and contains a Popover control.Menu appears over the host application
getProperty
method of the ResourceModel to provide a translated text for a given key, the getProperty method was the perfect place to alter the output.sap/ui/util/Storage
.Data stored in the local storage
sap.ui.define([
"sap/ui/model/resource/ResourceModel",
"sap/ui/util/Storage",
"./UserInterface"
], function (ResourceModel, LocalStorage, UserInterface ) {
"use strict";
var DeepResourceModel = ResourceModel.extend("com.sample.DeepTranslation.ResourceModel", {
status: { enabled: false, transform: "none" },
constructor: function (oData) {
ResourceModel.apply(this, arguments);
this.status = this.getStatus();
UserInterface.init(this);
},
getProperty: function( sPath ) {
let s = ResourceModel.prototype.getProperty.call( this, sPath );
if ( this.status.enabled ) {
this.injectGetText();
switch ( this.status.transform ) {
case "upper":
s = s.toUpperCase();
break;
case "hidenum":
s = s.replaceAll(/(\d{1,15}\.?\d{1,15})/g,"****");
break;
}
}
return s;
},
getStatus: function() {
let storage = new LocalStorage(LocalStorage.Type.local, "tr");
let storageKey = "status-"+this.oData.bundleName;
let status = JSON.parse(storage.get( storageKey )) || { "enabled": false };
return status;
},
saveStatus: function() {
let storage = new LocalStorage(LocalStorage.Type.local, "tr");
let storageKey = "status-"+this.oData.bundleName;
storage.put( storageKey, JSON.stringify( this.status ) );
},
setEnabled: function( isEnabled ) {
this.status.enabled = isEnabled;
this.saveStatus();
this.refresh(true);
},
setTransformation: function( transformation ) {
this.status.transform = transformation;
this.saveStatus();
this.refresh(true);
},
injectGetText: function() {
let oRB = this.getResourceBundle();
if ( oRB && !oRB.inheritedGetText ) {
oRB.inheritedGetText = oRB.getText;
oRB.getText = DeepResourceModel.getText.bind({ DeepResourceModel: this, bundle: oRB });
}
}
});
const KEYREF = new RegExp(/\(\(\((.*?)\)\)\)/, 'g');
DeepResourceModel.getText = function (sKey, aArgs, bIgnoreKeyFallback) {
let sTemplate = this.bundle.inheritedGetText(sKey, aArgs, bIgnoreKeyFallback);
let sTranslated = sTemplate;
if ( this.DeepResourceModel.status.enabled ) {
for (const captureGroup of sTemplate.matchAll(KEYREF)) {
let sub = this.bundle.getText(captureGroup[1], [], bIgnoreKeyFallback)
sTranslated = sTranslated.replace(captureGroup[0], sub);
}
}
return sTranslated
};
return DeepResourceModel;
});
sap.ui.define([
"sap/ui/core/Fragment",
"sap/ui/core/Popup"
], function( Fragment, Popup ) {
"use strict";
var UserInterface = {
_oPopup: null,
init: function( resourceModel ) {
document.addEventListener("keydown", this.handleKeypress.bind(this), { capture: true } );
this.resourceModel = resourceModel;
this.UI = new sap.ui.model.json.JSONModel( this.defineModelData() );
},
defineModelData: function() {
return {
enabled: false,
transform: "none"
}
},
handleKeypress: function( ev ) {
if ( ev.keyCode === 84 ) {
if ( ev.altKey && ev.ctrlKey && ev.shiftKey ) {
ev.stopImmediatePropagation();
this.openTranslationMenu();
}
}
},
openTranslationMenu: function() {
if ( !this._oPopup ) {
this._oPopup = new Popup(this, true, false, false );
}
if ( this._oPopup && !this._oPopup.isOpen() ) {
Fragment.load({
type: 'XML', definition: MENU_FRAGMENT,
controller: this,
id: "translation-frame-menu"
}).then(function( fragment ){
fragment.setModel( this.UI, "UI" );
this.UI.setProperty("/enabled", this.resourceModel.status.enabled );
this.UI.setProperty("/transform", this.resourceModel.status.transform );
this._oPopup.setContent( fragment );
this._oPopup.open(1, sap.ui.core.Popup.Dock.EndTop, sap.ui.core.Popup.Dock.EndTop, document, '-32 32', "none");
}.bind(this));
}
},
handleCloseMenu: function() {
if ( this._oPopup !== null ) {
this._oPopup.close(0);
}
},
handleSwitch: function( oEvent ) {
this.resourceModel.setEnabled( this.UI.getProperty("/enabled") );
},
handleTransformationChange: function( oEvent ) {
this.resourceModel.setTransformation( this.UI.getProperty("/transform") );
}
};
/* ==========================================================
* Inline XML fragment definition
* ========================================================== */
let MENU_FRAGMENT =
`<core:FragmentDefinition xmlns:html="http://www.w3.org/1999/xhtml" xmlns="sap.m" xmlns:core="sap.ui.core">
<Popover title="Translation Experiments" class="sapUiSizeCompact" contentMinWidth="28rem">
<VBox>
<InputListItem label="Local Translation Support:" class="sapUiTinyMargin">
<Switch state="{UI>/enabled}" change="handleSwitch"/>
</InputListItem>
<InputListItem label="Text transformation:" class="sapUiTinyMargin">
<Select selectedKey="{UI>/transform}" enabled="{UI>/enabled}" change="handleTransformationChange">
<core:Item key="none" text="None"/>
<core:Item key="upper" text="Upper case"/>
<core:Item key="hidenum" text="Hide numbers"/>
</Select>
</InputListItem>
</VBox>
<endButton>
<Button icon="sap-icon://decline" press="handleCloseMenu"/>
</endButton>
</Popover>
</core:FragmentDefinition>`;
return UserInterface;
});
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
9 | |
8 | |
5 | |
5 | |
4 | |
4 | |
4 | |
3 | |
3 | |
3 |