Hello everyone,
We needed to call SAP PI/PO RFC integration with Java according to a customer's need. I was able to call functions from SAP using JCo before, but I had not worked on triggering the SAP PI/PO RFC sender. After some research, I found out how to do it and I wanted to share it with you.
Before we write our Java code, we have a small but necessary step to do.
- First of all, you need to download the JCo driver for your operating system. It is important to review the installation.html page located in the javadoc folder in the driver archive you downloaded. Actions to be taken according to your operating system are written on the HTML page. (SAP JCo Page)
- You need to add "jco.allow_non_abap_partner" parameter to the VM parameter of SAP PI/PO instance. You can review this SNote showing how to add a parameter. (1729203 - Support for communication with external RFC server)
- Next step is create a RFC sender channel. You can create a standart RFC sender channel. But you have to enable Advanced Mode. Also you need to unchecked Verify Sender System parameter.

My channel looks like;
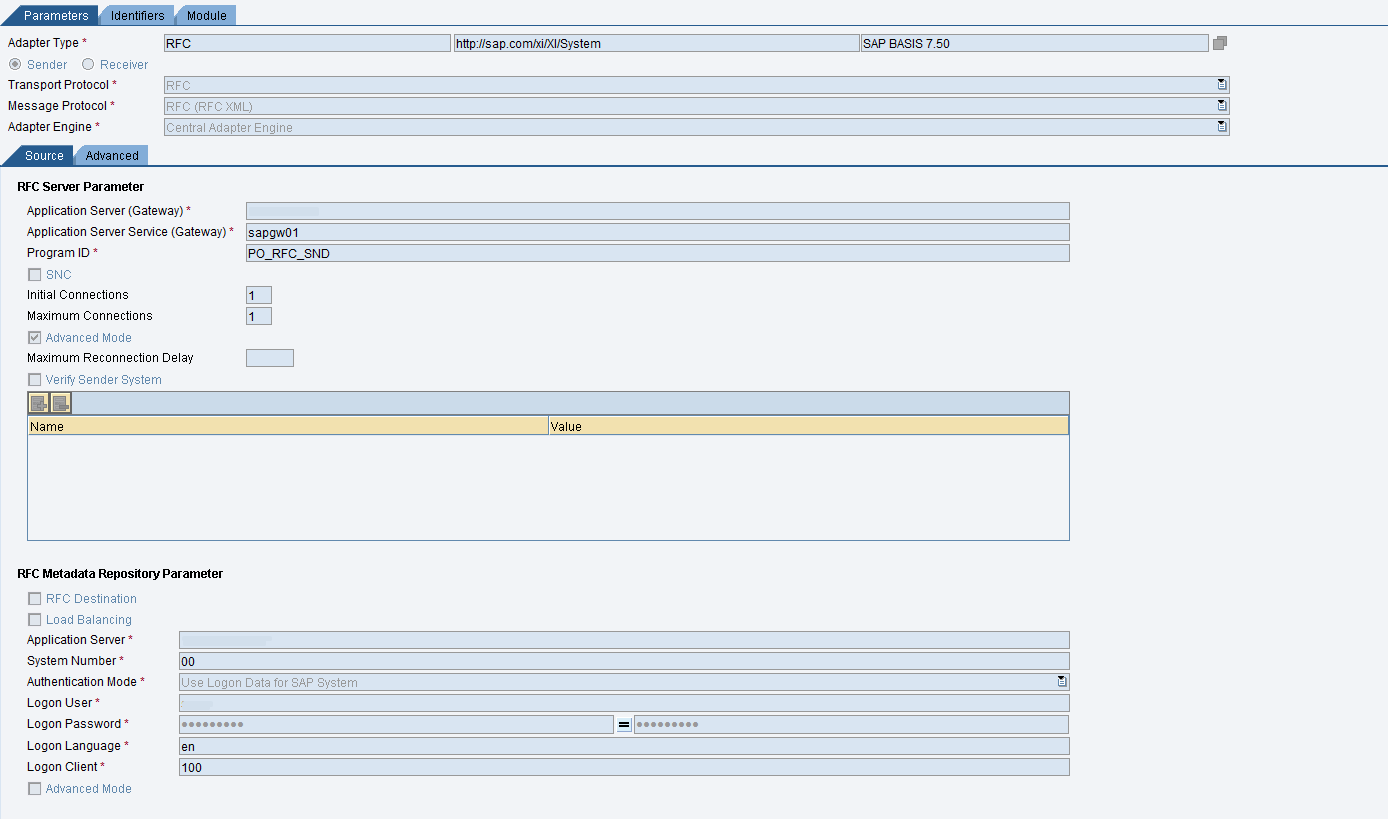
- Also we need to create an RFC integration. I imported a function to ESR from SAP which I will call from Java.

Then I created an ICO with that function.

If everything is okay, let's start!
First, we need to define
SAP.jcoDestination file for Repository. You can fill this template with your SAP system information for
SAP.jcoDestination file.
jco.client.ashost=sap_application_server_host
jco.client.sysnr=sap_system_number
jco.client.client=sap_system_client_number
jco.client.user=sap_user
jco.client.passwd=sap_user_password
jco.client.lang=sap_language
Then, we have to create
SAP_PO.jcoDestination file for communicate with SAP PI/PO. You can fill this template with your PO system information for
SAP_PO.jcoDestination file.
jco.client.type=E
jco.client.gwhost=your_po_host
jco.client.gwserv=your_po_gateway
jco.client.tpname=PO_RFC_SND
jco.destination.repository_destination=repository_file_name
The
jco.client.tpname parameter represents the Program ID parameter on the RFC sender channel. I write
PO_RFC_SND to the value of this parameter because I write
PO_RFC_SND to the Program ID value in the RFC channel.
The value of
jco.destination.repository_destination parameter must be SAP Repository file name. We defined the name of the file as
SAP.jcoDestination in this blog. Therefore, we write
SAP to the value of the jco.destination.repository_destination parameter.
After the files are created, your project folder should look like this. (
The .jcoDestination files must be in the same place as your project. )

Let's code!
First, you have to add sapjco3.jar as external library to your project. If you done, you can continue next step.
Define a JCoDestination variable. We have to write SAP_PO in
getDestination method. Because our PO destination configuration file name is
SAP_PO.jcoDestination. (If you named the file differently, use the name you typed. Don't forget! The file extension must be
.jcoDestination. )
JCoDestination dest = JCoDestinationManager.getDestination("SAP_PO");
We have to define JCoFunction variable. After that, we will use getFunction method with function name which we want to call. I will use "RFC_FUNCTION_SEARCH" function.
JCoFunction function = dest.getRepository().getFunction("RFC_FUNCTION_SEARCH");
Now, we need to set import parameters of RFC_FUNCTION_SEARCH function. You can see parameters of the function with SE37 tcode in SAP system.

I can see only FUNCNAME parameter is mandatory. I want to get all Z* named functions from SAP system therefore I assigned "Z*" to the FUNCNAME parameter.
function.getImportParameterList().setValue("FUNCNAME","Z*");
I am running the function.
function.execute(dest);
If you want to get result after execute the function, you can do with below code.
String result = function.getTableParameterList().toJSON();
System.out.println(result);
Result;

package com.example;
import com.sap.conn.jco.JCoDestination;
import com.sap.conn.jco.JCoDestinationManager;
import com.sap.conn.jco.JCoException;
import com.sap.conn.jco.JCoFunction;
import java.io.IOException;
public class Main {
public static void main(String[] args) throws IOException, JCoException {
// Define a JCoDestination variable. We have to write SAP_PO in getDestination method.
// Because our PO destination configuration file name is SAP_PO.jcoDestination.
// (If you named the file differently, use the name you typed. Don't forget! The file extension must be .jcoDestination. )
JCoDestination dest = JCoDestinationManager.getDestination("SAP_PO");
//We can define the function which we want to call. I will use "RFC_FUNCTION_SEARCH" function.
JCoFunction function = dest.getRepository().getFunction("RFC_FUNCTION_SEARCH");
//Now, we need to set import parameters of RFC_FUNCTION_SEARCH function.
// You can see parameters of the function with SE37 tcode in SAP system.
function.getImportParameterList().setValue("FUNCNAME","Z*");
//Execute the function
function.execute(dest);
//Print result
String result = function.getTableParameterList().toJSON();
System.out.println(result);
}
}
When you call the RFC with Java, you can see the message in Message Monitor.

Conclusion
In summary, with this blog you can call the RFC integrations you developed in PI/PO with Java using the JCO library. In addition, based on this example, you can also call the function from SAP without PI/PO. You can use the
SimpleCall.java example in the JCO file you downloaded.
Thank you for reading.