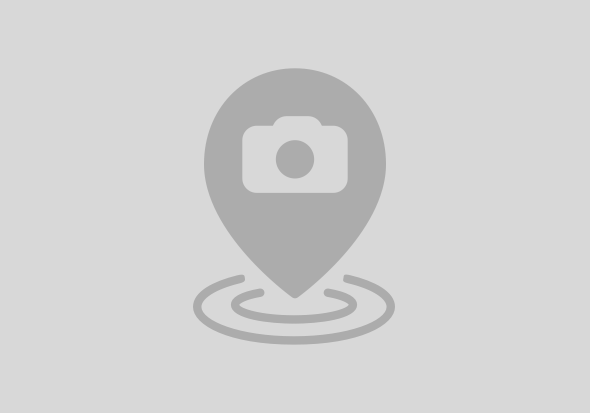
oAuth Flow (M2M)
REPORT zs4tf_oauth_call_blog.
START-OF-SELECTION.
PARAMETERS: tokenurl TYPE string LOWER CASE,
clientid TYPE string LOWER CASE,
clientsc TYPE string LOWER CASE,
no_auth AS CHECKBOX,
apiurl TYPE string LOWER CASE OBLIGATORY,
apikey TYPE string LOWER CASE OBLIGATORY,
method TYPE string OBLIGATORY DEFAULT 'GET',
body type string lower case.
IF no_auth NE 'X' .
* 1 POST OAUTH2.0 Call to get Token
cl_http_client=>create_by_url( EXPORTING url = tokenurl ssl_id = 'ANONYM'
IMPORTING client = DATA(lo_http_client_token) ) .
"adding headers with API Key for API Sandbox
lo_http_client_token->propertytype_logon_popup = 0.
lo_http_client_token->request->set_method( 'POST' ).
lo_http_client_token->request->set_header_fields( VALUE #(
( name = 'Accept' value = '*/*' )
( name = 'Content-Type' value = 'application/x-www-form-urlencoded' ) ) ) .
lo_http_client_token->request->set_form_fields( VALUE #(
( name = 'client_id' value = clientid )
( name = 'client_secret' value = clientsc )
( name = 'grant_type' value = 'client_credentials' ) ) ).
* Send / Receive Request
lo_http_client_token->send( EXCEPTIONS OTHERS = 1 ).
IF sy-subrc <> 0. MESSAGE ID sy-msgid TYPE sy-msgty NUMBER sy-msgno WITH sy-msgv1 sy-msgv2 sy-msgv3 sy-msgv4. ENDIF.
lo_http_client_token->receive( EXCEPTIONS OTHERS = 1 ).
IF sy-subrc > 0. MESSAGE ID sy-msgid TYPE sy-msgty NUMBER sy-msgno WITH sy-msgv1 sy-msgv2 sy-msgv3 sy-msgv4. ENDIF.
*Display result
lo_http_client_token->response->get_status( IMPORTING code = DATA(l_status_code) ).
IF l_status_code = 200.
DATA(rv_response) = lo_http_client_token->response->get_cdata( ) .
ELSE.
DATA lt_fields TYPE tihttpnvp .
lo_http_client_token->response->get_header_fields( CHANGING fields = lt_fields ).
cl_demo_output=>display_data( lt_fields ).
ENDIF.
cl_demo_output=>display_json( rv_response ) .
* Extract Access Token
DATA: BEGIN OF ls_token,
access_token TYPE string,
token_type TYPE string,
expires_in TYPE string,
scope TYPE string,
jti TYPE string,
END OF ls_token.
/ui2/cl_json=>deserialize( EXPORTING json = rv_response CHANGING data = ls_token ) .
ENDIF.
* 2 GET Call to API (Application Call)
CONCATENATE 'Bearer' ls_token-access_token INTO DATA(ls_access) SEPARATED BY space .
cl_http_client=>create_by_url( EXPORTING url = apiurl ssl_id = 'ANONYM' IMPORTING client = DATA(lo_http_client_api) ) .
"adding headers with API Key for API Sandbox
lo_http_client_api->propertytype_logon_popup = 0.
lo_http_client_api->request->set_method( method ). "here a POST or another method could be used as well depending on API
lo_http_client_api->request->set_header_fields( VALUE #(
( name = 'APIKey' value = apikey )
( name = 'DataServiceVersion' value = '2.0' )
( name = 'Accept' value = '*/*' )
( name = 'Content-Type' value = 'application/json' ) ) ).
IF no_auth NE 'X' .
lo_http_client_api->request->set_header_field( name = 'Authorization' value = ls_access ) .
ENDIF.
if not body is initial.
lo_http_client_api->request->set_cdata( body ) .
endif.
* Send / Receive Request
lo_http_client_api->send( EXCEPTIONS OTHERS = 1 ).
IF sy-subrc <> 0. MESSAGE ID sy-msgid TYPE sy-msgty NUMBER sy-msgno WITH sy-msgv1 sy-msgv2 sy-msgv3 sy-msgv4. ENDIF.
lo_http_client_api->receive( EXCEPTIONS OTHERS = 1 ).
IF sy-subrc > 0. MESSAGE ID sy-msgid TYPE sy-msgty NUMBER sy-msgno WITH sy-msgv1 sy-msgv2 sy-msgv3 sy-msgv4. ENDIF.
*Display result
lo_http_client_api->response->get_status( IMPORTING code = DATA(l_status_code_api) ).
IF l_status_code_api = 200.
DATA(rv_response_api) = lo_http_client_api->response->get_cdata( ) .
ELSE.
DATA lt_fields_api TYPE tihttpnvp .
lo_http_client_api->response->get_header_fields( CHANGING fields = lt_fields_api ).
cl_demo_output=>display_data( lt_fields_api ).
ENDIF.
cl_demo_output=>display_json( rv_response_api ) .
Report selection screen
Parameter | Value |
NO_AUTH | X |
APIURL | https://sandbox.api.sap.com/SAPCALM/calm-projects/v1/projects |
APIKEY | jzSnQuhsY1atvSDxJfeBk1LfWlsE69f0 |
METHOD | GET |
Parameter | Value |
NO_AUTH | X |
APIURL | https://sandbox.api.sap.com/spatialservices/geocoding/v1/geocode |
APIKEY | jzSnQuhsY1atvSDxJfeBk1LfWlsE69f0 |
METHOD | POST |
BODY | {"credentials": { "provider": "Here", "api_key": "SAP-KEY" }, "addresses": [ "Biel,Schweiz" ]} |
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
5 | |
2 | |
2 | |
2 | |
2 | |
2 | |
2 | |
1 | |
1 | |
1 |