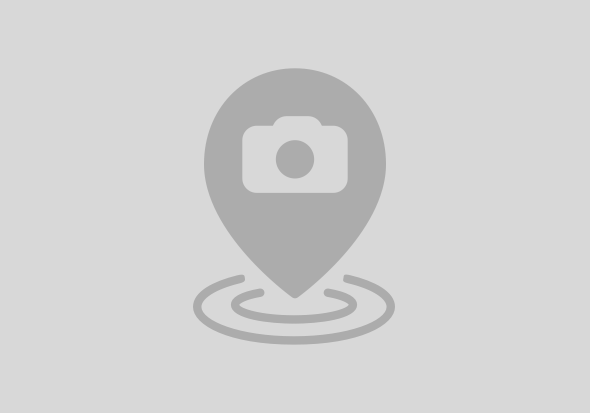
Exception Handling Architecture
boolean supports(Class clazz😞 this method is used to decide whether current error converter can process passed error, which type is indicated by clazz. Returning true means current error converter can process passed error.
void populate(final SOURCE source, final TARGET target😞 this method is used to convert passed source(error) to target (ErrorWsDTO) .
Error Converters and Supported Error Types
Logs Segment as logstack Is True
webservicescommons.resthandlerexceptionresolver.{extensionName}.{exceptionName}.status=401
webservicescommons.resthandlerexceptionresolver.{exceptionName}.status=403
webservicescommons.resthandlerexceptionresolver.DEFAULT.status=400
...
@Controller
...
public class ProductReviewController
{
...
@RequestMapping(value = "/{userId}/", method = RequestMethod.GET)
...
public ResponseEntity<Double> getAverageReviewRating(@Parameter(description = "customer id", required = true)
@PathVariable(value = "userId")
final String customerId)
{
final CustomerModel customer = (CustomerModel) userService.getUserForUID(customerId);
final Double averageReviewRating = productReviewService.getAverageReviewRating(customer);
return new ResponseEntity<Double>(averageReviewRating, HttpStatus.OK);
}
...
}
...
public class CustomerReviewException extends WebserviceException
{
public static final String NO_REVIEW = "noReviews";
private static final String TYPE = "ReviewRatingError";
private static final String SUBJECT_TYPE = "review";
public CustomerReviewException(final String message)
{
super(message);
}
public CustomerReviewException(final String message, final String reason)
{
super(message, reason);
}
public CustomerReviewException(final String message, final String reason, final Throwable cause)
{
super(message, reason, cause);
}
public CustomerReviewException(final String message, final String reason, final String subject)
{
super(message, reason, subject);
}
@Override
public String getType()
{
return TYPE;
}
@Override
public String getSubjectType()
{
return SUBJECT_TYPE;
}
}
CustomerReviewValidationData is used to represent validation error:
...
public class CustomerReviewValidationData implements Serializable
{
private static final long serialVersionUID = 1L;
private String message;
private String reason;
public CustomerReviewValidationData() { }
public CustomerReviewValidationData(final String reason, final String message)
{
this.reason = reason;
this.message = message;
}
public String getReason()
{
return reason;
}
public String getMessage()
{
return message;
}
}
CustomerReviewValidationDataList is used to represent a list of validation errors
...
public class CustomerReviewValidationDataList implements Serializable
{
private static final long serialVersionUID = 1L;
private List<CustomerReviewValidationData> customerReviewValidationDataList;
public CustomerReviewValidationDataList() { }
public void setCustomerReviewValidationDataList(final List<CustomerReviewValidationData> customerReviewValidationDataList)
{
this.customerReviewValidationDataList = customerReviewValidationDataList;
}
public List<CustomerReviewValidationData> getCustomerReviewValidationDataList()
{
return customerReviewValidationDataList;
}
}
CustomerReviewValidationErrorConverter is used to convert CustomerReviewValidationData to ErrorWsDTO
...
public class CustomerReviewValidationErrorConverter extends AbstractConverter<CustomerReviewValidationData, ErrorWsDTO>
{
private static final String TYPE = "customerReviewError";
private static final String SUBJECT_TYPE = "customerReview";
private static final String SUBJECT = "customerReviewNumber";
public CustomerReviewValidationErrorConverter() {}
@Override
public void populate(final CustomerReviewValidationData customerReviewValidationData, final ErrorWsDTO errorWsDTO)
{
errorWsDTO.setType(TYPE);
errorWsDTO.setSubjectType(SUBJECT_TYPE);
errorWsDTO.setSubject(SUBJECT);
errorWsDTO.setReason(customerReviewValidationData.getReason());
errorWsDTO.setMessage(customerReviewValidationData.getMessage());
}
}
CustomerReviewValidationListErrorConverter is used to convert a list of CustomerReviewValidationData to a list of ErrorWsDTO instances, it will delegate CustomerReviewValidationErrorConverter to finish concrete work.
...
public class CustomerReviewValidationListErrorConverter extends AbstractErrorConverter
{
private final CustomerReviewValidationErrorConverter customerReviewValidationErrorConverter;
public CustomerReviewValidationListErrorConverter(final CustomerReviewValidationErrorConverter converter)
{
this.customerReviewValidationErrorConverter = converter;
}
@Override
public boolean supports(final Class clazz)
{
return CustomerReviewValidationDataList.class.isAssignableFrom(clazz);
}
@Override
public void populate(final Object o, final List<ErrorWsDTO> webserviceErrorList)
{
final CustomerReviewValidationDataList customerReviewValidationDataList = (CustomerReviewValidationDataList) o;
webserviceErrorList.addAll(getCustomerReviewValidationErrorConverter()
.convertAll(customerReviewValidationDataList.getCustomerReviewValidationDataList()));
}
protected CustomerReviewValidationErrorConverter getCustomerReviewValidationErrorConverter()
{
return customerReviewValidationErrorConverter;
}
}
<alias alias="customerReviewValidationErrorConverter" name="defaultCustomerReviewValidationErrorConverter" />
<bean name="defaultCustomerReviewValidationErrorConverter" class="my.commerce.eduocc.errors.converters.CustomerReviewValidationErrorConverter">
<property name="targetClass" value="de.hybris.platform.webservicescommons.dto.error.ErrorWsDTO"/>
</bean>
<alias alias="customerReviewValidationListErrorConverter" name="defaultCustomerReviewValidationListErrorConverter" />
<bean name="defaultCustomerReviewValidationListErrorConverter" class="my.commerce.eduocc.errors.converters.CustomerReviewValidationListErrorConverter">
<constructor-arg ref="customerReviewValidationErrorConverter" />
</bean>
<alias alias="webserviceErrorFactory" name="defaultWebserviceErrorFactory" />
<bean id="defaultWebserviceErrorFactory" class="de.hybris.platform.webservicescommons.errors.factory.impl.DefaultWebserviceErrorFactory">
<property name="converters">
<list>
<ref bean="validationErrorConverter" />
<ref bean="cartVoucherValidationListErrorConverter" />
<ref bean="cartModificationDataListErrorConverter" />
<ref bean="customerReviewValidationListErrorConverter" />
<ref bean="webserviceExceptionConverter" />
<ref bean="exceptionConverter" />
</list>
</property>
</bean>
...
@Controller
...
public class ProductReviewController
{
...
@RequestMapping(value = "/{userId}/", method = RequestMethod.GET)
...
public ResponseEntity<Double> getAverageReviewRating(@Parameter(description = "customer id", required = true)
@PathVariable(value = "userId")
final String customerId)
{
final CustomerModel customer = (CustomerModel) userService.getUserForUID(customerId);
final Double averageReviewRating = productReviewService.getAverageReviewRating(customer);
if ((averageReviewRating != null) && (averageReviewRating > 0))
{
final List<CustomerReviewValidationData> list = validateCustomerReviewData(customer);
if (!list.isEmpty())
{
final CustomerReviewValidationDataList crValidationDataList = new CustomerReviewValidationDataList();
crValidationDataList.setCustomerReviewValidationDataList(list);
throw new WebserviceValidationException(crValidationDataList);
}
return new ResponseEntity<Double>(averageReviewRating, HttpStatus.OK);
}
else
{
//return new ResponseEntity<Double>(HttpStatus.NO_CONTENT);
throw new CustomerReviewException("no average review rating", CustomerReviewException.NO_REVIEW,
"the average review rating is null");
}
}
...
}
No Customer Review
Two Validation Errors
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
2 | |
1 | |
1 | |
1 | |
1 | |
1 | |
1 | |
1 | |
1 | |
1 |