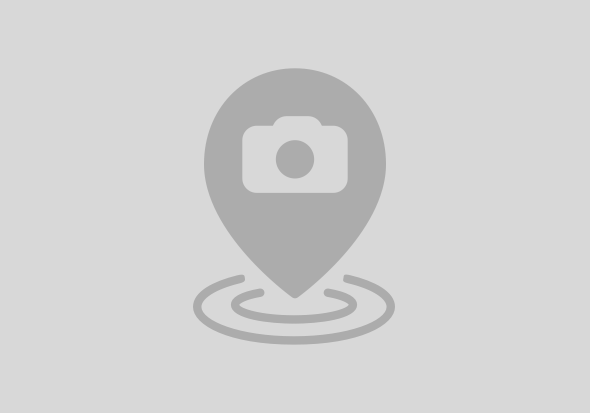
pfunctions
|--- pom.xml
|--- src
| |--- pom.xml
| |--- src
| |--- main
| |--- java
| |--- ... pfunctions source code
|--- assembly
|--- pom.xml
|--- commerce
| |--- pom.xml
| |--- main
| |--- assembly
| |--- all-pfunctions-jar.xml
|--- sme
|--- pom.xml
src
subproject compiles the pfunctions code, while the assembly
subproject generates the pfunctions assemblies. The sme
and commerce
subprojects under the assembly
subproject are creating the assemblies respectively for the Solution Modeling Environment and SAP Commerce.pfunctions/pom.xml
file contains the following content:<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>mygroup</groupId>
<artifactId>pfunctions-parent</artifactId>
<version>1.0.0</version>
<packaging>pom</packaging>
<name>My pFunctions</name>
<organization>
<name>My Organization</name>
</organization>
<properties>
<java.version>1.8</java.version>
<ssc.release>3.2</ssc.release>
<ssc.version>${ssc.release}.21</ssc.version>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<maven.build.timestamp.format>yyyyMMddHHmmssSSS</maven.build.timestamp.format>
<build.version>${project.version}.${maven.build.timestamp}</build.version>
</properties>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>com.sap.custdev.projects.fbs.slc</groupId>
<artifactId>ssc</artifactId>
<version>${ssc.version}</version>
<scope>provided</scope>
</dependency>
</dependencies>
</dependencyManagement>
<modules>
<module>src</module>
<module>assembly</module>
</modules>
</project>
pfunctions/src/pom.xml
file contains the following content:<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>mygroup</groupId>
<artifactId>pfunctions-parent</artifactId>
<version>1.0.0</version>
<relativePath>..</relativePath>
</parent>
<artifactId>pfunctions</artifactId>
<version>1.0.0</version>
<packaging>jar</packaging>
<name>My pFunctions Sources</name>
<dependencies>
<dependency>
<groupId>com.sap.custdev.projects.fbs.slc</groupId>
<artifactId>ssc</artifactId>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.0</version>
<configuration>
<encoding>${project.build.sourceEncoding}</encoding>
<source>${java.version}</source>
<target>${java.version}</target>
</configuration>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-jar-plugin</artifactId>
<version>2.1</version>
<configuration>
<archive>
<addMavenDescriptor>false</addMavenDescriptor>
<manifest>
<addDefaultImplementationEntries>true</addDefaultImplementationEntries>
<addDefaultSpecificationEntries>true</addDefaultSpecificationEntries>
</manifest>
<manifestEntries>
<Implementation-Version>${build.version}</Implementation-Version>
</manifestEntries>
</archive>
</configuration>
</plugin>
</plugins>
</build>
</project>
pfunctions/assembly/pom.xml
file contains the following content:<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>mygroup</groupId>
<artifactId>pfunctions-parent</artifactId>
<version>1.0.0</version>
<relativePath>..</relativePath>
</parent>
<artifactId>pfunctions-assembly</artifactId>
<packaging>pom</packaging>
<name>My pFunctions Assembly POM</name>
<description>Assemble the pFunctions into different packages to be used in different environments</description>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>${project.groupId}</groupId>
<artifactId>pfunctions</artifactId>
<version>${project.version}</version>
</dependency>
</dependencies>
</dependencyManagement>
<build>
<pluginManagement>
<plugins>
<plugin>
<artifactId>maven-eclipse-plugin</artifactId>
<version>3.1.0</version>
</plugin>
</plugins>
</pluginManagement>
</build>
<modules>
<module>commerce</module>
<module>sme</module>
</modules>
</project>
pfunctions/assembly/commerce/pom.xml
file contains the following content:<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>mygroup</groupId>
<artifactId>pfunctions-assembly</artifactId>
<version>1.0.0</version>
<relativePath>..</relativePath>
</parent>
<artifactId>pfunctions-assembly-commerce</artifactId>
<version>1.0.0</version>
<packaging>jar</packaging>
<name>My pFunctions Assembly for SAP Commerce</name>
<dependencies>
<dependency>
<groupId>${project.groupId}</groupId>
<artifactId>pfunctions</artifactId>
</dependency>
</dependencies>
<build>
<finalName>mypfunctions</finalName>
<plugins>
<plugin>
<artifactId>maven-assembly-plugin</artifactId>
<configuration>
<appendAssemblyId>false</appendAssemblyId>
<descriptors>
<descriptor>src/main/assembly/all-pfunctions-jars.xml</descriptor>
</descriptors>
<archive>
<addMavenDescriptor>false</addMavenDescriptor>
<manifest>
<addDefaultImplementationEntries>true</addDefaultImplementationEntries>
<addDefaultSpecificationEntries>true</addDefaultSpecificationEntries>
</manifest>
<manifestEntries>
<Implementation-Version>${build.version}</Implementation-Version>
</manifestEntries>
</archive>
</configuration>
<executions>
<execution>
<id>assemble-pfunctions-jar</id>
<phase>package</phase>
<goals>
<goal>single</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
</project>
all-pfunctions-jars.xml
. Here is the content of this file.<?xml version="1.0" encoding="UTF-8"?>
<assembly xmlns="http://maven.apache.org/ASSEMBLY/2.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/ASSEMBLY/2.0.0 http://maven.apache.org/xsd/assembly-2.0.0.xsd">
<id>all-pfunctions-jars</id>
<formats>
<format>jar</format>
</formats>
<includeBaseDirectory>false</includeBaseDirectory>
<dependencySets>
<dependencySet>
<unpack>true</unpack>
<unpackOptions>
<excludes>
<exclude>META-INF/**</exclude>
</excludes>
</unpackOptions>
<useTransitiveDependencies>true</useTransitiveDependencies>
</dependencySet>
</dependencySets>
</assembly>
pfunctions/assembly/sme/pom.xml
file contains the following content:<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>mygroup</groupId>
<artifactId>pfunctions-assembly</artifactId>
<version>1.0.0</version>
<relativePath>..</relativePath>
</parent>
<artifactId>pfunctions-assembly-sme</artifactId>
<version>1.0.0</version>
<packaging>bundle</packaging>
<name>My pFunctions Assembly for Eclipse/SME</name>
<dependencies>
<dependency>
<groupId>${project.groupId}</groupId>
<artifactId>pfunctions-assembly-commerce</artifactId>
<version>${project.version}</version>
<scope>compile</scope>
</dependency>
</dependencies>
<build>
<finalName>mypfunctions_${project.version}</finalName>
<plugins>
<plugin>
<groupId>org.apache.felix</groupId>
<artifactId>maven-bundle-plugin</artifactId>
<version>2.5.0</version>
<extensions>true</extensions>
<configuration>
<archive>
<addMavenDescriptor>false</addMavenDescriptor>
<manifest>
<addDefaultImplementationEntries>true</addDefaultImplementationEntries>
<addDefaultSpecificationEntries>true</addDefaultSpecificationEntries>
</manifest>
<manifestEntries>
<Implementation-Version>${build.version}</Implementation-Version>
</manifestEntries>
</archive>
<manifestLocation>${project.build.outputDirectory}/META-INF</manifestLocation>
<instructions>
<Bundle-Name>My pFunctions</Bundle-Name>
<Bundle-SymbolicName>mypfunctions;singleton=true</Bundle-SymbolicName>
<Bundle-Vendor>My Organization</Bundle-Vendor>
<Bundle-Version>${project.version}</Bundle-Version>
<Bundle-RequiredExecutionEnvironment>JavaSE-1.6</Bundle-RequiredExecutionEnvironment>
<Bundle-ActivationPolicy>lazy</Bundle-ActivationPolicy>
<Export-Package>com.sap.sce.user,com.sap.sce.user.helpers,com.sap.sce.engine.ddb,com.sap.front.base</Export-Package>
<Require-Bundle>com.sap.custdev.projects.fbs.slc.ejb-ipc;bundle-version="${ssc.release}";resolution:=optional</Require-Bundle>
<Eclipse-RegisterBuddy>com.sap.custdev.projects.fbs.slc.ejb-ipc</Eclipse-RegisterBuddy>
<Embed-Dependency>*</Embed-Dependency>
<Embed-Transitive>false</Embed-Transitive>
<Embed-StripGroup>true</Embed-StripGroup>
<Embed-StripVersion>false</Embed-StripVersion>
</instructions>
</configuration>
</plugin>
</plugins>
</build>
</project>
<instructions>
element.mvn install:install-file \
-Dfile=<SSC JAR file path> \
-DgroupId=com.sap.custdev.projects.fbs.slc \
-DartifactId=ssc \
-Dpackaging=jar \
-Dversion=<SSC JAR version (e.g. 3.2.21)>
mvn clean install
. It will build the pfunctions code, create the assemblies and add these assemblies to your local Maven repository. If you want to grab the assemblies, go into the target
folder of each assembly subproject to find the JAR.mvn -Dssc.version=<your SSC version> clean install
. Ensure this version of Solution Sales Configuration is either available in your organization private Maven repository or in your local Maven repository.pfunctions/pom.xml
file:<?xml version=""?>
<project ...>
[...]
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-engine</artifactId>
<version>5.7.0</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.mockito</groupId>
<artifactId>mockito-core</artifactId>
<version>3.5.13</version>
<scope>test</scope>
</dependency>
<!-- needed by Solution Sales Configuration library to execute unit tests -->
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>LATEST</version>
<scope>test</scope>
</dependency>
[...]
</dependencies>
</dependencyManagement>
[...]
</project>
pfunctions
|--- src
|--- src
|--- main
|--- test
|--- java
|--- ... pfunctions unit test code
src
subproject to run the JUnit tests by adding the following content to the pfunctions/src/pom.xml
file.<?xml version="1.0"?>
<project ...>
[...]
<dependencies>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-engine</artifactId>
</dependency>
<dependency>
<groupId>org.mockito</groupId>
<artifactId>mockito-core</artifactId>
</dependency>
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
</dependency>
</dependencies>
[...]
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>3.0.0-M3</version>
<configuration>
<includes>
<include>**/*Test.java</include>
<include>**/Test*.java</include>
<include>**/*Tests.java</include>
<include>**/*TestCase.java</include>
<include>**/*_TEST.java</include>
<include>**/TEST_*.java</include>
</includes>
<classpathDependencyExcludes>
<classpathDependencyExclude>com.sap.custdev.projects.fbs.slc:ejb-ipc</classpathDependencyExclude>
</classpathDependencyExcludes>
</configuration>
</plugin>
</plugins>
</build>
[...]
</project>
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
2 | |
1 | |
1 | |
1 | |
1 | |
1 | |
1 | |
1 | |
1 | |
1 |