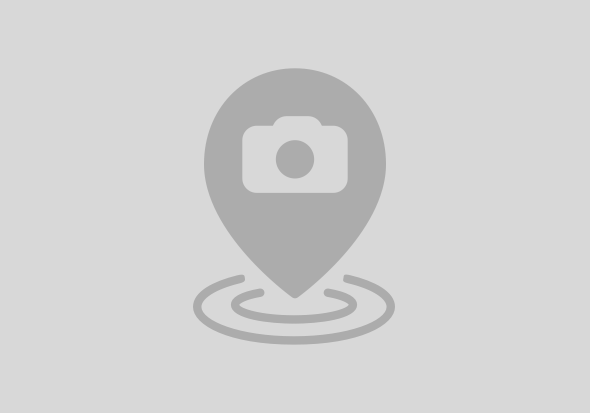
SELECT vbeln,posnr, matnr, kwmeng, vrkme
FROM vbap
INTO TABLE @DATA(lt_vbap)
WHERE vbeln IN @s_vbeln.
IF sy-subrc = 0.
LOOP AT lt_vbap INTO DATA(ls_vbap).
CALL FUNCTION 'CONVERSION_EXIT_MATN1_OUTPUT'
EXPORTING
input = ls_vbap-matnr
IMPORTING
output = ls_vbap-matnr.
APPEND VALUE #( converted_matnr = ls_vbap-matnr ) TO lt_display.
ENDLOOP.
cl_demo_output=>display( lt_display ).
ENDIF.
CLASS lcl_conversion_routine DEFINITION.
PUBLIC SECTION.
METHODS:
matnr_input IMPORTING iv_matnr TYPE matnr
RETURNING VALUE(rv_matnr) TYPE matnr,
matnr_output IMPORTING iv_matnr TYPE matnr
RETURNING VALUE(rv_matnr) TYPE matnr,
menge_output IMPORTING iv_menge TYPE any
iv_meins TYPE meins
RETURNING VALUE(rv_menge) TYPE char17,
meins_output IMPORTING iv_meins TYPE meins
RETURNING VALUE(rv_meins) TYPE meins.
ENDCLASS.
CLASS lcl_conversion_routine IMPLEMENTATION.
METHOD matnr_input.
*& Material Conversion External to Internal.
CALL FUNCTION 'CONVERSION_EXIT_MATN1_INPUT'
EXPORTING
input = iv_matnr
IMPORTING
output = rv_matnr
EXCEPTIONS
length_error = 1
OTHERS = 2 ##FM_SUBRC_OK.
ENDMETHOD.
METHOD matnr_output.
*& Material Conversion Internal to External.
CALL FUNCTION 'CONVERSION_EXIT_MATN1_OUTPUT'
EXPORTING
input = iv_matnr
IMPORTING
output = rv_matnr ##FM_SUBRC_OK.
ENDMETHOD.
METHOD meins_output.
*& Unit of Measure Internal to External Format.
CALL FUNCTION 'CONVERSION_EXIT_CUNIT_OUTPUT'
EXPORTING
input = iv_meins
IMPORTING
output = rv_meins
EXCEPTIONS
unit_not_found = 1
OTHERS = 2 ##FM_SUBRC_OK.
ENDMETHOD.
METHOD menge_output.
*& Quantity Internal to External Format.
WRITE iv_menge TO rv_menge UNIT iv_meins.
ENDMETHOD.
ENDCLASS.
TYPES: BEGIN OF ty_display,
unconverted_matnr TYPE text30,
converted_matnr TYPE text30,
unconverted_menge TYPE text30,
converted_menge TYPE text30,
unconverted_meins TYPE text30,
converted_meins TYPE text30,
END OF ty_display.
DATA: lt_display TYPE TABLE OF ty_display.
SELECT vbeln,posnr, matnr, kwmeng, vrkme
FROM vbap
INTO TABLE @DATA(lt_vbap)
WHERE vbeln IN @s_vbeln.
IF sy-subrc = 0.
lt_display = VALUE #( LET lo_conversion = NEW lcl_conversion_routine( ) IN
FOR ls_vbap IN lt_vbap
( unconverted_matnr = ls_vbap-matnr
unconverted_menge = ls_vbap-kwmeng
unconverted_meins = ls_vbap-vrkme
converted_matnr = lo_conversion->matnr_output( iv_matnr = ls_vbap-matnr )
converted_menge = lo_conversion->menge_output( iv_menge = ls_vbap-kwmeng
iv_meins = ls_vbap-vrkme )
converted_meins = lo_conversion->meins_output( iv_meins = ls_vbap-vrkme ) ) ).
lt_display = VALUE #( LET lt_external_values = lt_display
lo_conversion = NEW lcl_conversion_routine( ) IN
BASE lt_display
FOR ls_external_value IN lt_external_values
( unconverted_matnr = ls_external_value-converted_matnr
converted_matnr = lo_conversion->matnr_input( iv_matnr = CONV matnr( ls_external_value-converted_matnr ) ) ) ).
cl_demo_output=>display( lt_display ).
ENDIF.
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
2 | |
1 | |
1 | |
1 | |
1 | |
1 | |
1 | |
1 | |
1 | |
1 |